Note
Click here to download the full example code
Gaussian Process Prior¶
Hello world
import numpy as np
import tensorflow as tf
import tensorflow_probability as tfp
import matplotlib.pyplot as plt
# shortcuts
tfd = tfp.distributions
kernels = tfp.math.psd_kernels
# constants
num_features = 1 # dimensionality
num_index_points = 256 # nbr of index points
num_samples = 8
x_min, x_max = -5.0, 5.0
X_grid = np.linspace(x_min, x_max, num_index_points).reshape(-1, num_features)
seed = 23 # set random seed for reproducibility
random_state = np.random.RandomState(seed)
kernel = kernels.ExponentiatedQuadratic()
Kernel profile¶
The exponentiated quadratic kernel is stationary. That is, \(k(x, x') = k(x, 0)\) for all \(x, x'\).
fig, ax = plt.subplots()
ax.plot(X_grid, kernel.apply(X_grid, np.zeros((1, num_features))))
ax.set_xlabel(r'$x$')
ax.set_ylabel(r'$k(x, 0)$')
plt.show()
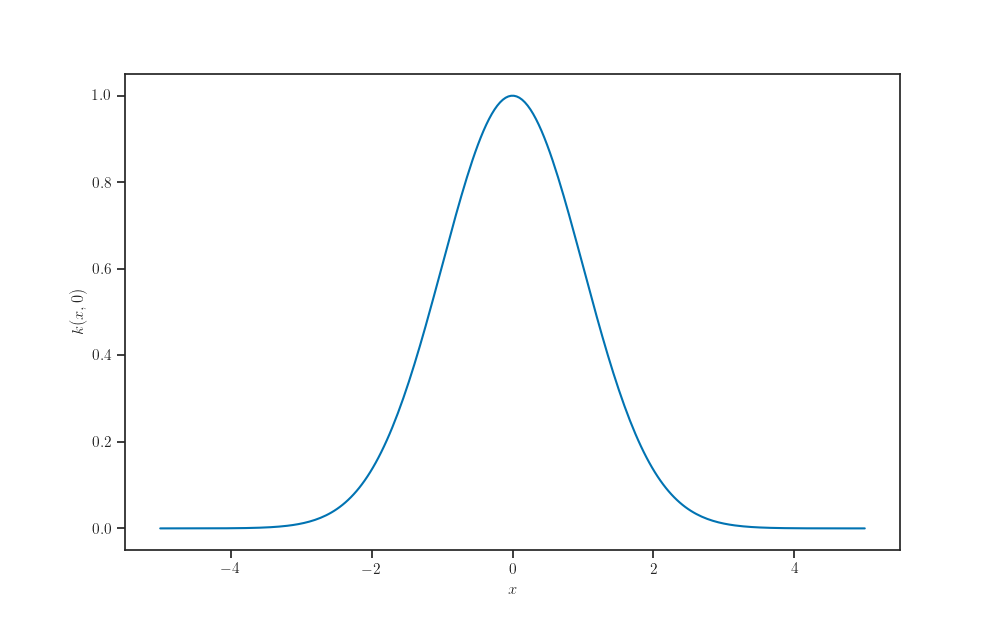
Kernel matrix¶
fig, ax = plt.subplots()
ax.pcolormesh(*np.broadcast_arrays(X_grid, X_grid.T),
kernel.matrix(X_grid, X_grid), cmap="cividis")
ax.invert_yaxis()
ax.set_xlabel(r'$x$')
ax.set_ylabel(r'$x$')
plt.show()
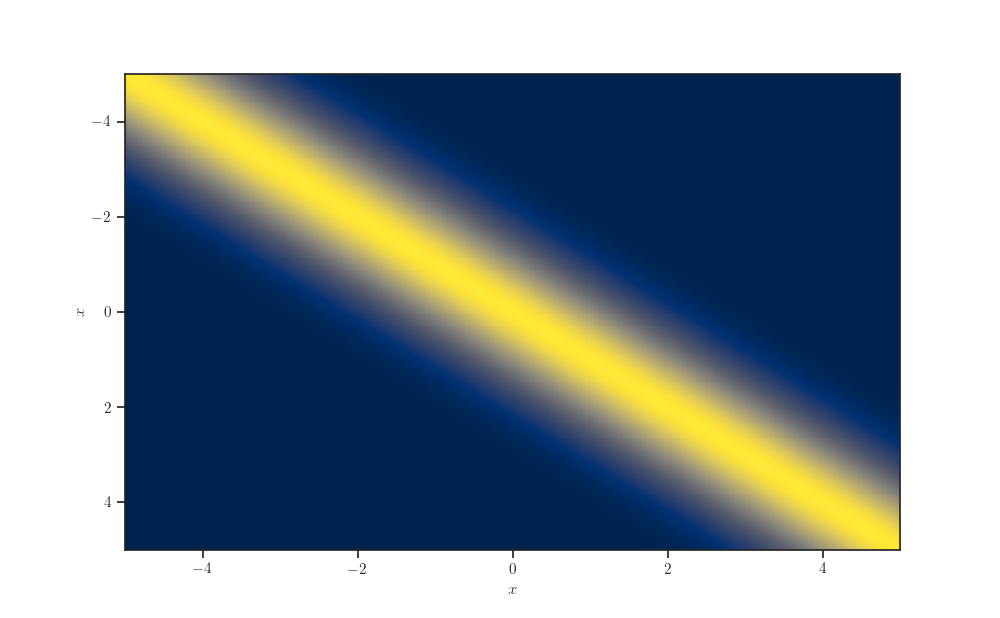
Out:
/usr/src/app/examples/gaussian_processes/plot_prior.py:57: MatplotlibDeprecationWarning: shading='flat' when X and Y have the same dimensions as C is deprecated since 3.3. Either specify the corners of the quadrilaterals with X and Y, or pass shading='auto', 'nearest' or 'gouraud', or set rcParams['pcolor.shading']. This will become an error two minor releases later.
kernel.matrix(X_grid, X_grid), cmap="cividis")
Prior samples¶
gp = tfd.GaussianProcess(kernel=kernel, index_points=X_grid)
samples = gp.sample(num_samples, seed=seed)
fig, ax = plt.subplots()
ax.plot(X_grid, samples.numpy().T)
ax.set_xlabel(r'$x$')
ax.set_ylabel(r'$f(x)$')
ax.set_title(r'Draws of $f(x)$ from GP prior')
plt.show()
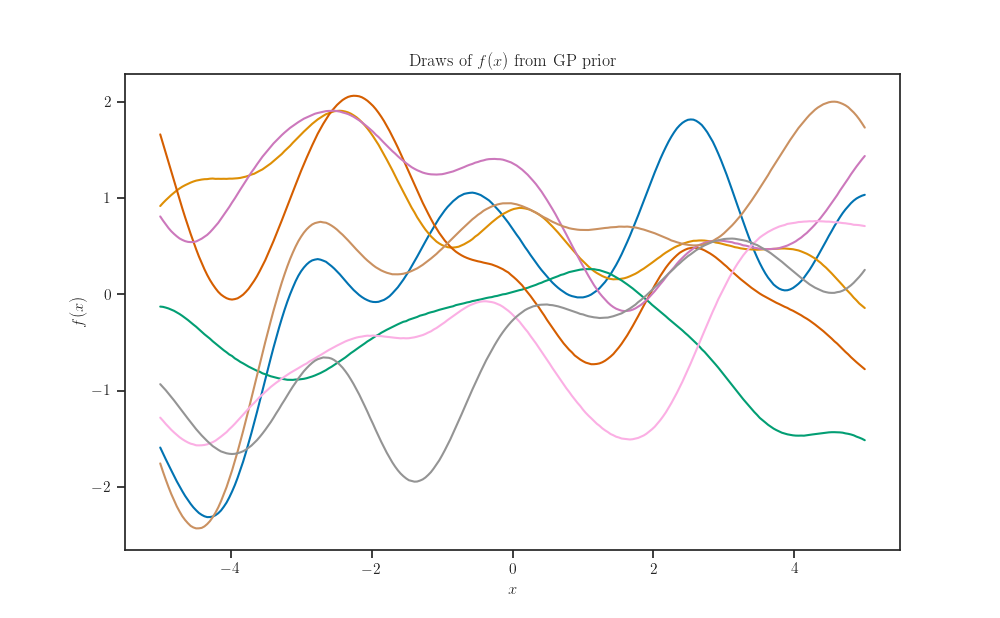
Total running time of the script: ( 0 minutes 1.068 seconds)