Note
Click here to download the full example code
Cryptocurrency Exchange Orderbook¶
A snapshot of an orderbook on Binance, a popular cryptocurrency exchange, for a specified symbol (trading pair).
Calls the Binance API endpoint using
requests
.Visualizes the result using
seaborn
.
import requests
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
pair = ("ETH", "AUD")
symbol = "".join(pair)
binwidth = 5.0
r = requests.get("https://api.binance.com/api/v3/depth", params=dict(symbol=symbol))
results = r.json()
results
Out:
{'lastUpdateId': 9471556, 'bids': [['931.19000000', '1.46128000'], ['931.18000000', '2.31947000'], ['930.58000000', '1.40948000'], ['930.57000000', '2.23727000'], ['929.99000000', '1.86266000'], ['929.36000000', '1.93694000'], ['928.78000000', '1.67087000'], ['927.96000000', '2.24815000'], ['927.27000000', '4.92350000'], ['926.73000000', '8.20849000'], ['926.05000000', '8.47277000'], ['925.91000000', '0.13450000'], ['925.37000000', '13.04865000'], ['924.44000000', '0.17750000'], ['924.43000000', '14.27661000'], ['924.08000000', '0.01083000'], ['923.96000000', '0.01440000'], ['923.35000000', '0.11400000'], ['923.34000000', '14.20232000'], ['922.37000000', '0.80400000'], ['922.29000000', '15.86952000'], ['921.49000000', '12.62321000'], ['920.97000000', '0.13450000'], ['920.56000000', '13.99541000'], ['919.87000000', '3.36000000'], ['919.74000000', '16.60342000'], ['918.64000000', '16.67119000'], ['917.72000000', '13.80404000'], ['916.31000000', '19.21281000'], ['916.04000000', '0.13450000'], ['916.01000000', '0.01092000'], ['915.52000000', '0.01440000'], ['915.30000000', '18.88199000'], ['914.89000000', '2.18605000'], ['913.89000000', '2.66000000'], ['911.10000000', '0.13450000'], ['908.84000000', '0.11004000'], ['908.00000000', '0.92296000'], ['907.15000000', '0.01440000'], ['907.00000000', '1.00000000'], ['906.16000000', '0.13450000'], ['906.00000000', '1.00000000'], ['905.00000000', '1.00000000'], ['904.00000000', '1.00000000'], ['903.00000000', '1.00000000'], ['902.00000000', '1.00000000'], ['901.22000000', '0.13450000'], ['901.00000000', '1.00000000'], ['900.00000000', '1.11111000'], ['899.00000000', '1.11234000'], ['898.85000000', '0.01440000'], ['898.00000000', '1.11358000'], ['897.63000000', '0.02284000'], ['897.00000000', '1.11482000'], ['896.28000000', '0.13450000'], ['896.00000000', '1.11607000'], ['895.84000000', '0.01173000'], ['895.51000000', '0.11168000'], ['895.00000000', '3.35194000'], ['894.14000000', '0.05592000'], ['894.00000000', '1.11856000'], ['893.00000000', '1.11982000'], ['892.00000000', '1.12107000'], ['891.34000000', '0.13450000'], ['891.00000000', '1.50626000'], ['890.64000000', '0.01440000'], ['889.10000000', '0.05624000'], ['889.00000000', '0.14624000'], ['886.40000000', '0.13450000'], ['885.00000000', '0.61265000'], ['884.33000000', '0.11308000'], ['882.50000000', '0.01440000'], ['881.46000000', '0.13450000'], ['880.00000000', '2.15911000'], ['876.53000000', '0.13450000'], ['874.43000000', '0.01440000'], ['871.59000000', '0.09150000'], ['866.65000000', '0.13450000'], ['866.43000000', '0.01440000'], ['863.00000000', '0.50000000'], ['861.71000000', '0.13450000'], ['858.51000000', '0.01440000'], ['856.77000000', '0.20676000'], ['856.66000000', '1.00000000'], ['851.83000000', '0.13450000'], ['851.62000000', '0.04719000'], ['851.00000000', '1.17508000'], ['850.66000000', '0.01440000'], ['850.00000000', '4.50000000'], ['848.06000000', '0.10891000'], ['846.89000000', '0.13450000'], ['846.73000000', '0.24069000'], ['842.89000000', '0.01440000'], ['841.95000000', '0.13450000'], ['841.12000000', '0.06374000'], ['840.00000000', '0.02348000'], ['837.18000000', '0.01764000'], ['837.02000000', '0.13450000'], ['836.71000000', '0.07396000'], ['835.18000000', '0.01440000']], 'asks': [['933.37000000', '3.94990000'], ['933.38000000', '6.26967000'], ['933.87000000', '2.09180000'], ['934.68000000', '0.05687000'], ['934.70000000', '1.83513000'], ['935.56000000', '0.07036000'], ['935.79000000', '0.13450000'], ['935.83000000', '0.50300000'], ['935.91000000', '11.01958000'], ['937.08000000', '9.20276000'], ['937.31000000', '0.16211000'], ['938.25000000', '3.29000000'], ['938.37000000', '0.11379000'], ['938.38000000', '12.14573000'], ['939.61000000', '5.66696000'], ['939.62000000', '0.42513000'], ['939.63000000', '12.11089000'], ['940.00000000', '3.00000000'], ['940.73000000', '0.13450000'], ['941.04000000', '14.68920000'], ['941.09000000', '0.01440000'], ['942.44000000', '39.15809000'], ['943.61000000', '37.45843000'], ['944.98000000', '28.44212000'], ['945.67000000', '0.13450000'], ['946.17000000', '0.01586000'], ['946.58000000', '32.70477000'], ['948.00000000', '2.69000000'], ['948.17000000', '35.18319000'], ['948.38000000', '0.01082000'], ['949.37000000', '35.02374000'], ['950.00000000', '0.67450000'], ['950.08000000', '0.14554000'], ['950.61000000', '0.13450000'], ['950.93000000', '27.33046000'], ['952.22000000', '31.54570000'], ['952.56000000', '1.18798000'], ['953.28000000', '0.01092000'], ['953.84000000', '35.62965000'], ['955.00000000', '2.05671000'], ['955.11000000', '38.84809000'], ['955.55000000', '0.13450000'], ['956.64000000', '25.25448000'], ['958.00000000', '0.10009000'], ['958.14000000', '26.22958000'], ['959.63000000', '28.79329000'], ['960.48000000', '0.13450000'], ['960.83000000', '38.55577000'], ['962.35000000', '26.47788000'], ['963.87000000', '26.58899000'], ['965.20000000', '31.03763000'], ['965.42000000', '0.13450000'], ['966.37000000', '29.97439000'], ['967.70000000', '27.32985000'], ['969.08000000', '35.26248000'], ['970.00000000', '55.00000000'], ['970.36000000', '0.13450000'], ['970.49000000', '27.41209000'], ['972.08000000', '28.28142000'], ['973.24000000', '36.09121000'], ['974.70000000', '28.74431000'], ['975.30000000', '0.13450000'], ['976.41000000', '33.91686000'], ['977.90000000', '32.34841000'], ['979.52000000', '28.97009000'], ['980.24000000', '0.13450000'], ['981.24000000', '31.74056000'], ['982.56000000', '28.91449000'], ['985.18000000', '0.13450000'], ['989.64000000', '0.01092000'], ['990.12000000', '0.13450000'], ['995.06000000', '0.13450000'], ['997.01000000', '0.01003000'], ['1000.00000000', '39.30288000'], ['1008.00000000', '0.10008000'], ['1020.00000000', '24.58905000'], ['1050.00000000', '5.87756000'], ['1052.70000000', '8.00040000'], ['1080.00000000', '2.03144000'], ['1200.00000000', '0.22387000'], ['1288.00000000', '25.65367000'], ['1388.00000000', '0.15017000'], ['1500.00000000', '6.85012000'], ['2000.00000000', '1.56084000'], ['3590.00000000', '1.00000000'], ['4000.00000000', '2.00000000'], ['4284.00000000', '8.62951000']]}
frames = []
for kind in ["bids", "asks"]:
frame = pd.DataFrame(data=results[kind],
columns=["price", "amount"],
dtype=float).assign(kind=kind)
frames.append(frame)
data = pd.concat(frames, axis="index", sort=True)
data
fig, ax = plt.subplots()
sns.scatterplot(x='amount', y='price', hue='kind', data=data, ax=ax)
ax.set_xlabel(f"Amount ({pair[0]})")
ax.set_ylabel(f"Price ({pair[1]})")
ax.set_xscale("log")
plt.show()
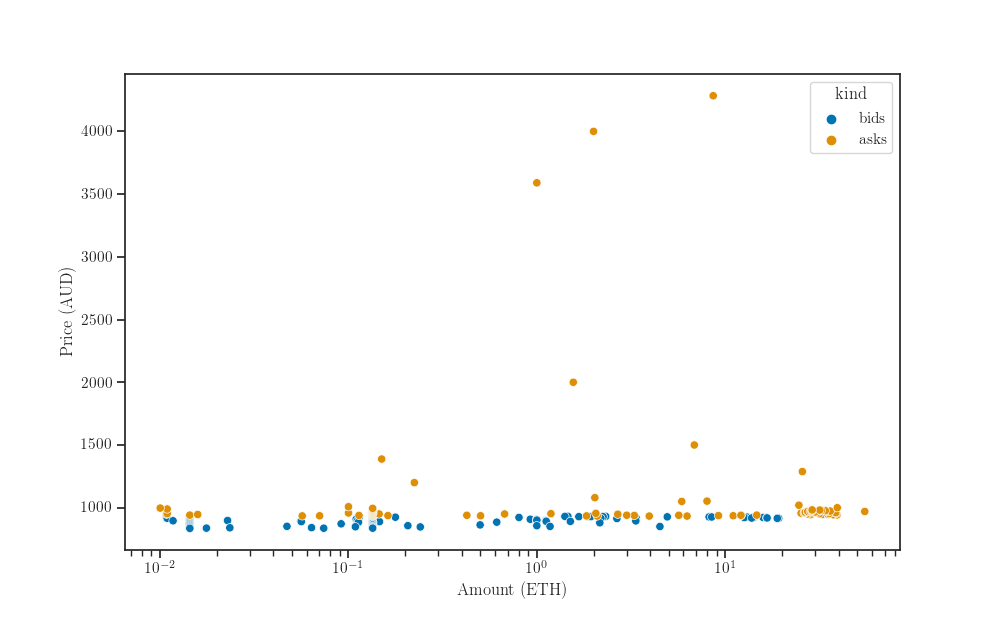
fig, ax = plt.subplots()
sns.histplot(x='price', hue='kind', binwidth=binwidth, data=data, ax=ax)
ax.set_xlabel(f"Price ({pair[1]})")
plt.show()
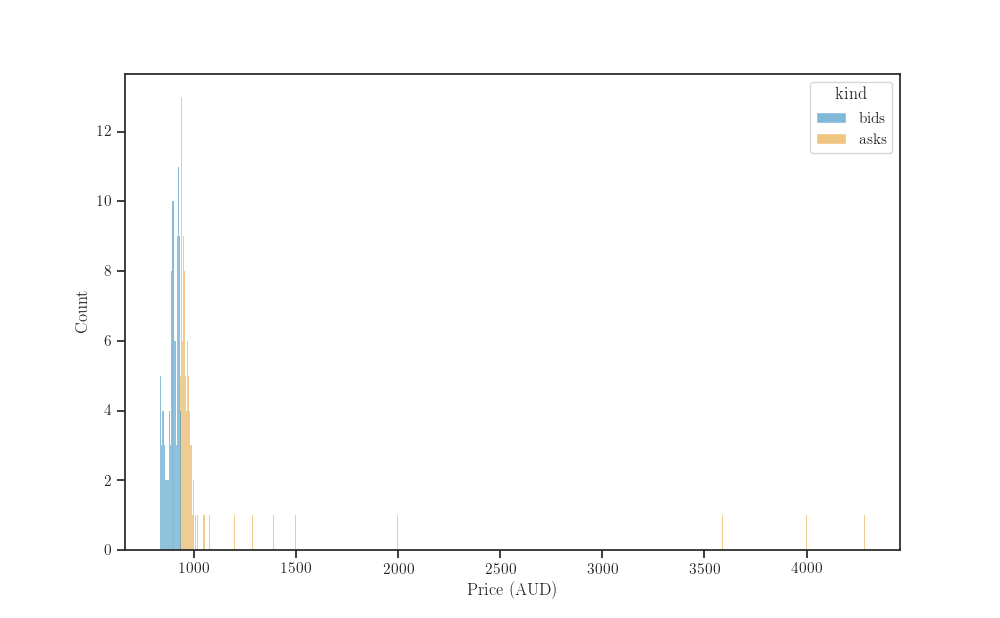
Weighted by amount.
fig, ax = plt.subplots()
sns.histplot(x='price', weights='amount', hue='kind', binwidth=binwidth,
data=data, ax=ax)
ax.set_xlabel(f"Price ({pair[1]})")
ax.set_ylabel(f"Amount ({pair[0]})")
plt.show()
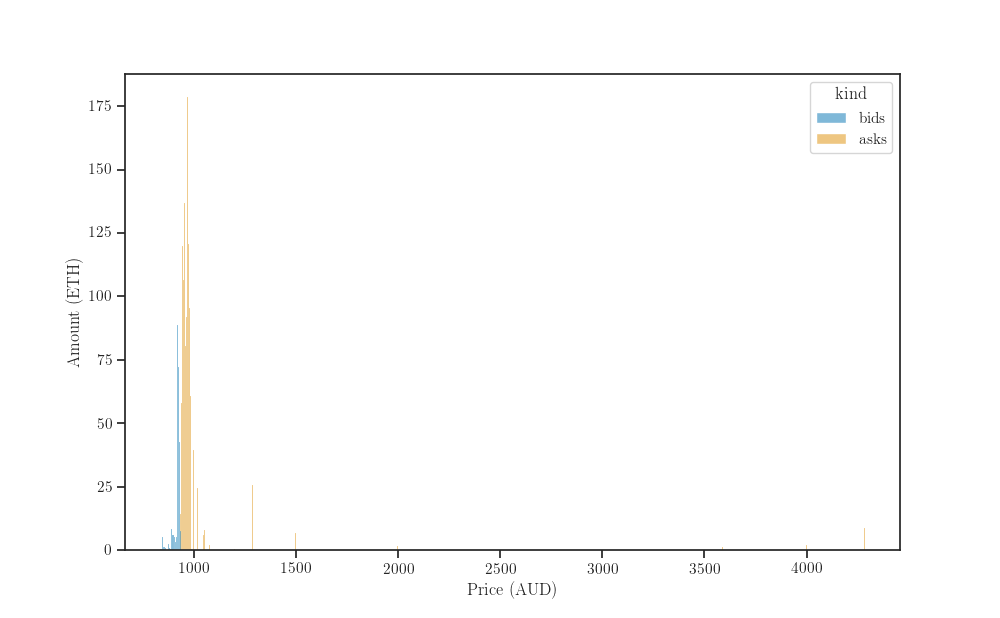
Total running time of the script: ( 0 minutes 5.222 seconds)